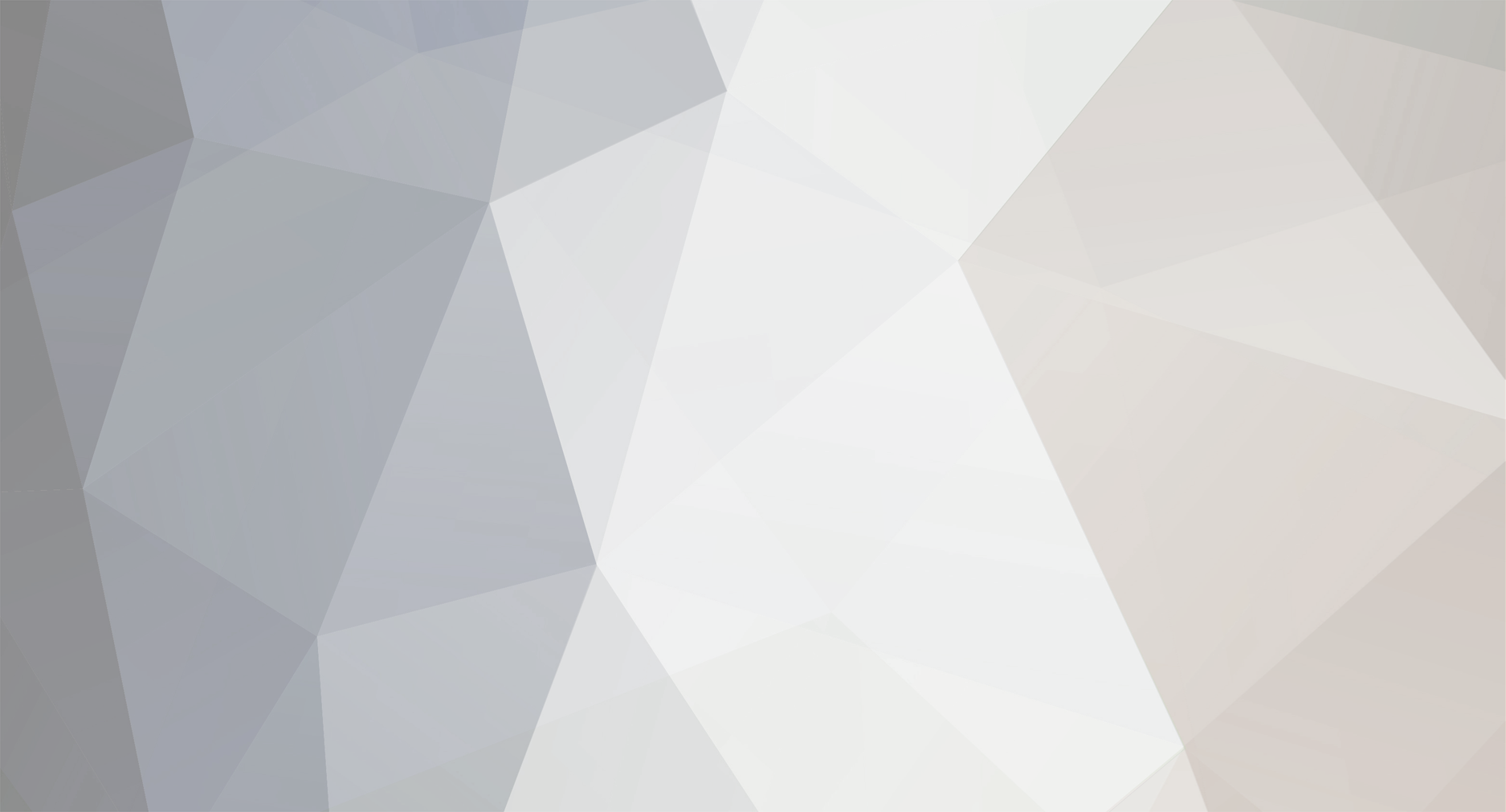
Miguel Barrera
Member-
Posts
663 -
Joined
-
Last visited
Content Type
Profiles
Forums
Events
Articles
Marionette
Store
Everything posted by Miguel Barrera
-
But in order to apply textures, don't you need Renderworks?
-
The color index refers to the number of colors in a palette. You should have a utility that can draw the active palette on a layer and if you export this chart to vectorscript it will write each color index with the corresponding RGB color values. As far as I know, only the first 16 index colors in the vw classic palette were fixed/reserved but the rest could be any color so you could create your own color palettes
-
Integers (short int) have a value range of -32,768 to 32,767 = 16 bits = 2 bytes whereas, Longint (long int) have a range of -2,147,483,648 to 2,147,483,647 = 32 bits = 4 bytes. For most purposes a short integer will be adequate but if the result of an operation is also a short and the value falls outside the limits of the integer, the result will be truncated to the lower/upper limits. This is why Trunc is declared as a LongInt. If the real value is 100,000.736 and it is assigned to a short Integer, the value will be truncated to 32,767 but if it is assigned to a LongInt then it will be 100,000 as expected. Moreover, rgb colors have a range of 0 to 65,535 and are declared as LongInt to hold the upper values but in reality it is an unsigned short integer meaning it does not have negative values. As for DIV and MOD, they are still part of VS but they are operands for integer Division and Remainder.
-
calculate arc center from polyline arc segment
Miguel Barrera replied to Hannes L's topic in Vectorscript
The following code makes use of vectors to calculate points which I think is simpler than using other methods. Note: 1. It is not bug free since there are special situations that must be handled before calling GetArcCenter. 2. Vectors can be displayed also as x,y,z but I chose the original vector notation v[1],v[2],v[3] to distinguish them from 2D/3D points. PROCEDURE GetPLineGeom; CONST kCORNER = 0; kBEZIER = 1; kCUBSPL = 2; kARCRAD = 3; VAR pLineHdl: HANDLE; vtxPt,pt1,pt3,arcCtr: POINT; i,vxTot,vxType: INTEGER; arcRad1,arcRad2,arcRad3: REAL; PROCEDURE GetArcCenter(vtxRad: REAL; VAR vtx1,vtx2,vtx3,ctrPt: POINT); VAR prpPt1,prpPt3: POINT; parLn,OnLn: BOOLEAN; tanLng,arcAng: REAL; vec1,vec2,prpVec1,prpVec2: VECTOR; BEGIN {vectors along tangent line} vec1[1]:= vtx1.x - vtx2.x; vec1[2]:= vtx1.y - vtx2.y; vec2[1]:= vtx3.x - vtx2.x; vec2[2]:= vtx3.y - vtx2.y; {This code segment will get the arc ends} {calculate length from vertex pt to the arc end} arcAng:= AngBVec(vec1,vec2); tanLng:= vtxRad / (Tan(Deg2Rad(arcAng/2))); {resize tangent vectors} vec1:= tanLng * UnitVec(vec1); vec2:= tanLng * UnitVec(vec2); {calculate the arc end points} vtx1.x:= vtx2.x + vec1[1]; vtx1.y:= vtx2.y + vec1[2]; vtx3.x:= vtx2.x + vec2[1]; vtx3.y:= vtx2.y + vec2[2]; {This will get the center} {get perpendicular vector to tangent line} prpVec1:= Perp(vec1); prpVec2:= Perp(vec2); {create points passing thru the end points} prpPt1.x:= vtx1.x + prpVec1[1]; prpPt1.y:= vtx1.y + prpVec1[2]; prpPt3.x:= vtx3.x + prpVec2[1]; prpPt3.y:= vtx3.y + prpVec2[2]; {calculate the intersection of the lines from the arc end points = arc center} LineLineIntersection(vtx1,prpPt1,vtx3,prpPt3,parLn,OnLn,ctrPt); END; BEGIN pLineHdl:= FSActLayer; IF (pLineHdl <> NIL) & (GetType(pLineHdl) = 21) THEN BEGIN vxTot:= GetVertNum(pLineHdl); FOR i:= 1 TO vxTot DO BEGIN GetPolylineVertex(pLineHdl,i,vtxPt.x,vtxPt.y,vxType,arcRad2); CASE vxType OF kCORNER: BEGIN WriteLn('Corner Pt ',i:0,' = ',Num2StrF(vtxPt.x),Num2StrF(vtxPt.y)); Tab(1); WriteLn('radius = ',Num2StrF(arcRad2)); END; kBEZIER: BEGIN WriteLn('Bezier Pt ',i:0,' = ',Num2StrF(vtxPt.x),Num2StrF(vtxPt.y)); Tab(1); WriteLn('radius = ',Num2StrF(arcRad2)); END; kCUBSPL: BEGIN WriteLn('Cubic Pt ',i:0,' = ',Num2StrF(vtxPt.x),Num2StrF(vtxPt.y)); Tab(1); WriteLn('radius = ',Num2StrF(arcRad2)); END; kARCRAD: BEGIN {Need to check if index is within array bounds} GetPolylineVertex(pLineHdl,i-1,pt1.x,pt1.y,vxType,arcRad1); GetPolylineVertex(pLineHdl,i+1,pt3.x,pt3.y,vxType,arcRad3); {If arcRad2 = 0, need to calculate max. radius} GetArcCenter(arcRad2,pt1,vtxPt,pt3,arcCtr); Locus(pt1.x,pt1.y); Locus(pt3.x,pt3.y); Locus(arcCtr.x,arcCtr.y); WriteLn('Arc Pt ',i:0,' = ',Num2StrF(vtxPt.x),Num2StrF(vtxPt.y)); Tab(1); WriteLn('radius = ',Num2StrF(arcRad2),'; center = ',Num2StrF(arcCtr.x),Num2StrF(arcCtr.y)); END; END; END; END; END; Run(GetPLineGeom); -
select object by class and hide/unhide
Miguel Barrera replied to zuken86's topic in General Discussion
Workspace Editor->Menus and under 'New Menu' drag the 'Separator' to the menu layout on the right box. -
VW will always crash if you attemp to open another window while a dialog is executing, so even the debugger will not work because it is also a window. The safest method I found is to "Write" the results to the output or open file.
-
calculate arc center from polyline arc segment
Miguel Barrera replied to Hannes L's topic in Vectorscript
You can use vector geometry to get the center mathematically. 1. Get the vectors between pt1 & pt2 and pt2 & pt3. 2. Get the perpendicular vectors perpV1 & perpV2. 3. Get the perpendicular lines passing thru pt1 & pt3 4. Use LineLineIntersection procedure to find the arc center. -
I believe that offsets for splines and beziers are converted to polygons instead of polylines. So, how close they match a true offset depends on the resolution of the conversion in the preferences.
-
I follow most of the code except the last condition where it could be blank. If xArray is filled consecutively with all the fixtures in the document, then a blank record would be the end of the array, so why would you need to fill another record? How are you adding data to the xArray? Are the fixtures the same PIO?
-
It might be easier to merge the shape files in Arcview and then import to VW. The alternative would be a script to merge them in VW
-
how to set the location of a symbol from text
Miguel Barrera replied to David Bengali's topic in Vectorscript
My preference would be to use IF ValidNumStr('your string',dim) THEN rather than distanceX:=Str2Num('your string'); -
One parameter to contol two different plugin objects?
Miguel Barrera replied to MaxStudio's topic in Vectorscript
All pio's already have a record with the same name as the pio. You only need to add a hidden parameter to the pio's list. The alternative method as discussed before, which is more complicated, is to use the event handling feature where VS tells you which parameter has changed. -
One parameter to contol two different plugin objects?
Miguel Barrera replied to MaxStudio's topic in Vectorscript
To expand on Dieter logic, you need to compare previous with current width to reset the other object and prevent it from looping back and forth. IF prevWdt <> currWdt THEN BEGIN SetRField(obj2Hdl,obj2Name,obj2FieldPrev,Num2Str(4,currWdt)); SetRField(obj2Hdl,obj2Name,obj2FieldCurr,Num2Str(4,currWdt)); ResetObject(obj2Hdl); END; If the updating is only one-way then you only need to set the new width on the other object SetRField(obj2Hdl,obj2Name,obj2FieldCurr,Num2Str(4,currWdt)); ResetObject(obj2Hdl); -
PROCEDURE AlertInform(text:STRING; advice:STRING; minorAlert:BOOLEAN); with minorAlert = TRUE, it will display the message on the status bar.
-
I am not very clear on this either. I do know that the knots are created with the nurbs curve and setting them to those values ensures it is a straight line. BTW the nurbs code was copied from exporting VS. When a file is not open for writing, these calls go to the Output.txt file and VS errors to the ErrorOut.txt file in your user folder. Since you have to drill down many folders to get to them, I have links on the desktop for easy access.
-
You can create different types of path objects, including your own path pio's, which are defined by name in the active workspace. FUNCTION DrawPipe(className: STRING; pipeDia,pipeThk: REAL; begPt,endPt: VECTOR): HANDLE; VAR midPt: VECTOR; outerRad,innerRad: REAL; outerHdl,innerHdl,profHdl,pathHdl,pipeHdl: HANDLE; BEGIN outerRad:= pipeDia / 2; innerRad:= outerRad - pipeThk; {Create group profile object} BeginGroup; ArcByCenter(0,0,outerRad,#0d,#360d); outerHdl:= LNewObj; IF pipeThk > 0 THEN BEGIN ArcByCenter(0,0,innerRad,#0d,#360d); innerHdl:= LNewObj; IF NOT AddHole(outerHdl,innerHdl) THEN WriteLn('Could not add hole'); DelObject(innerHdl); END; EndGroup; SetClass(outerHdl,className); profHdl:= LNewObj; midPt.x:= (begPt.x + endPt.x) / 2; midPt.y:= (begPt.y + endPt.y) / 2; midPt.z:= (begPt.z + endPt.z) / 2; {Create nurbs line path object} pathHdl:= CreateNurbsCurve(begPt.x,begPt.y,begPt.z,TRUE,1); IF pathHdl <> NIL THEN BEGIN AddVertex3D(pathHdl,midPt.x,midPt.y,midPt.z); AddVertex3D(pathHdl,endPt.x,endPt.y,endPt.z); NurbsSetWeight(pathHdl,0,0,1); NurbsSetWeight(pathHdl,0,1,1); NurbsSetKnot(pathHdl,0,0,0); NurbsSetKnot(pathHdl,0,1,0); NurbsSetKnot(pathHdl,0,2,1); NurbsSetKnot(pathHdl,0,3,1); END ELSE WriteLn('Could not create path'); pipeHdl:= CreateCustomObjectPath('Extrude Along Path', pathHdl, profHdl); IF (pipeHdl <> NIL) THEN BEGIN SetRField(pipeHdl,'Extrude Along Path', 'Scale', 'Uniform'); SetRField(pipeHdl,'Extrude Along Path','Factor','1'); SetClass(pipeHdl,className); END; DrawPipe:= pipeHdl; END; This function will draw a linear pipe
-
To set the graphic attributes with SetClass, you need to apply it to the profile object(s). The profile object should also be a group even if it contains only one object. If you use CreateCustomObjectPath('Extrude Along Path',MyPathH,MyProfileH); as Pat suggested and I also use, you do not need to delete the path and profile objects; they become part of the extrude. To see how an extrude could be coded, draw an extrude manually with classes or any other attributes and then export to vectorscript.
-
In older versions, you can also set the crop object to a class and toggle its visibility with the viewport classes
-
1. Check total number of existing classes 2. Create class by calling NameClass(className) 3. Get class total again 4. If both totals are the same, the class exists and vs sets it as the active class. If second total increases, then the class does not exist and the new class becomes the active one.
-
Get rid of attribute options in pio's!
Miguel Barrera replied to Dieter @ DWorks's question in Wishlist - Feature and Content Requests
I think you can still serve both users. If the pio has colors by class selected in the attributes palette, it can have precedence over the parts, which all will display the pio class colors. If the pio color by class is not selected, by choosing any other color, then the parts can display the assigned class colors for each one. -
Get rid of attribute options in pio's!
Miguel Barrera replied to Dieter @ DWorks's question in Wishlist - Feature and Content Requests
I understand the limitations of the VW stock pio's, such as windows and doors, but you were also making reference to developing your own pio's. I do not see such limitation or adding to much extra code when you are programming your own. You, the programmer, set the rules of how your pio will handle the objects classes and attributes. -
Get rid of attribute options in pio's!
Miguel Barrera replied to Dieter @ DWorks's question in Wishlist - Feature and Content Requests
I do both and to me it is just one line of code; setting each to a class. If desired, the attributes of the parts can be changed from the classes dialog. -
Get rid of attribute options in pio's!
Miguel Barrera replied to Dieter @ DWorks's question in Wishlist - Feature and Content Requests
This one I do not get. Can you elaborate on how it will be easier and faster? -
hyper text and hyper linking
Miguel Barrera replied to Dom9362's question in Wishlist - Feature and Content Requests
This can be done with VS. Not only can you link text but also link any object to a file, such as a specification, or a web page.