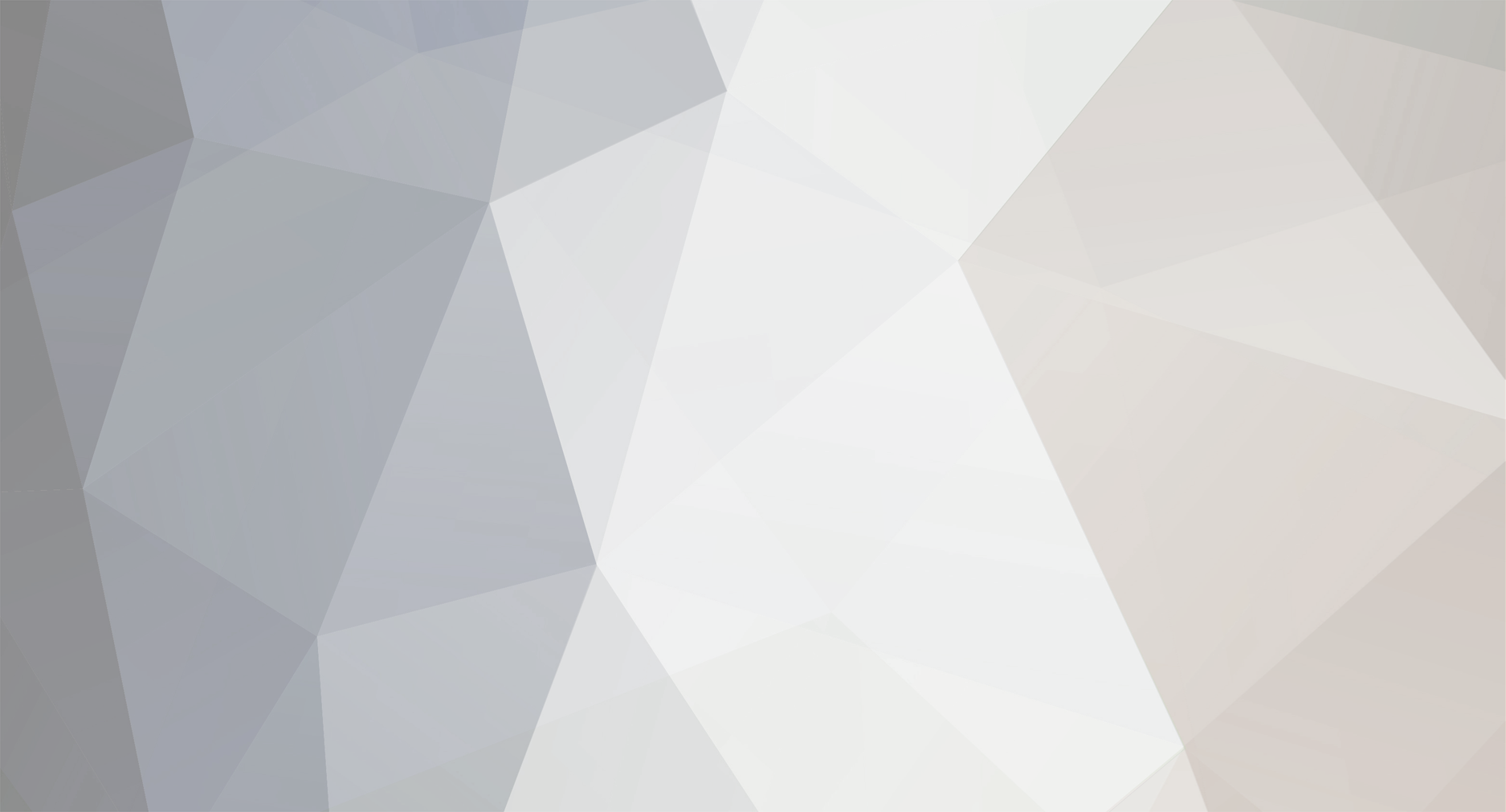
Will
Member-
Posts
159 -
Joined
-
Last visited
Content Type
Profiles
Forums
Events
Articles
Marionette
Store
Everything posted by Will
-
OK so I'm writing a pretty complicated script and I don't want it all in one file. I have a whole bunch of functions which are very simple and just wrap the original vs function. I want to put these in a file called wrappers.py. Like this: import vs import vso.base import vso def angToVec(angle, distance): v = vs.Ang2Vec(angle, distance) return Pt( v[0], v[1] ) pass def penLoc(): p = vs.PenLoc() return Pt( p[0], p[1] ) pass def moveTo(pt): vs.MoveTo(pt.xy()) pass def lineTo(pt): vs.LineTo(pt.xy()) pass The directory structure of my package looks like this: vso/ __init__py base.py wrappers.py To test this I have a script in a script palette in vector works which contains simply import vso If the above functions are in __init__.py, they work. If I paste them into wrappers.py and import them into __init__.py like this for wrappers import * I get the error Traceback (most recent call last): File "", line 4, in File "/Users/will/vso/__init__.py, line 75, in polar v = angToVec(ang, dist) File "/Users/will/vso/wrappers.py", line 5, in angToVec NameError: global name vs is not defined. Even though, as you can see from the contents of my wrappers.py file above, I am clearly importing the vs module. Python definitely allows importing the same module into multiple files. Is there some limitation in the Vectorworks implementation where I can only use import vs once per script? Any other clues about whats going on here? This (and python modules in general) is driving me slightly mad... I wish you had chosen Ruby, its much cuter... :.-(
-
Remove Layer Stacking Order Column
Will replied to Kevin McAllister's question in Wishlist - Feature and Content Requests
+1 -
Restore After Crash
Will replied to Tom Klaber's question in Wishlist - Feature and Content Requests
+1 for auto restore + MAX_INT for no crashes -
DOH! You're right of course. I'm used to Ruby which lets you leave them off.
-
vs.ArcByCenter(0, 0, 10.0, 0, 360) vs.SetLWByClass(vs.LNewObj) Gives this traceback I'm assuming that's a bug?
-
Detect out of date references instantly
Will posted a question in Wishlist - Feature and Content Requests
It would be great if out of date references could be detected as soon as the referenced file is updated on disk and optionally automatically updated straight away. This can be easily done on OSX by utilising the File System Events API I don't know how easy it is on Windows, but hey we didn't get multithreaded hidden line at them same time they did. -
I like to use hatches and tiles to show materials in elevation as vector lines on drawings rather than renderings as this keeps the file size of the resulting PDFs below the 5mb limit for submitting planning applications online and makes it easy to email drawings to clients. I am using a 3D workflow and hatches seem be generated as vectors in elevations when using the hidden line view or unshaded polygon render mode in a viewport in a sheet layer. However, tiles don't work. So when I need to use tiles for, say, stone walls in elevations, I have to draw polygons for these manually in annotation layers of sheet viewports. Both hatches and tiles don't show in unshaded polygon view in model layers, however. Feature request: In hidden line and polygon views I should have the option to see hatches and tiles in section viewports generated as vectors so that the resulting output file sizes are manageable. I would also like to see these when working in model layers. Download demo file
-
Can I update a section viewport from a script? Whats the function I need to call? Thanks, Will
-
PROCEDURE Main; VAR selectedPoly, lastSlate:HANDLE; pX, pY, pZ, outZ:REAL; offsetX, offsetY, offsetZ : REAL; rotationXAngle, rotationYAngle, rotationZAngle : REAL; throwawayBool:BOOLEAN; pt, beg_pt, END_pt, mat_org_pt, v:VECTOR; FUNCTION CheckObjCallback(h : HANDLE) : BOOLEAN; VAR objType:INTEGER; BEGIN {GetTypeN Returns the type index of the referenced planar or screen object. Rectangle 3 RECT. Polygon 5 POLY} objType := GetTypeN(h); IF ((objType = 3) OR (objType = 5)) THEN CheckObjCallback := true ELSE CheckObjCallback := false; END; BEGIN {Ask user to select a Poly} TrackObject(CheckObjCallback, selectedPoly, pX, pY, pZ); {Stuff the point at which poly is selected into a VECTOR} pt.x := pX; pt.y := pY; pt.z := pZ; {Get the matrix which transforms the Poly's plane from Document X-Y to the 3D plane which it is drawn on} throwawayBool := GetEntityMatrix(selectedPoly, offsetX, offsetY, offsetZ, rotationXAngle, rotationYAngle, rotationZAngle); Message('Found Poly: handle:', selectedPoly, ' Entity Matrix: x: ', offsetX, ' y: ', offsetY, ' z: ', offsetZ, ' ', rotationXAngle, ' ', rotationYAngle, ' ', rotationZAngle); {Stuff the orgin of the Poly's plane into a VECTOR} beg_pt.x := offsetX; beg_pt.y := offsetY; beg_pt.z := offsetZ; {Make a VECTOR which is 1 unit long on the X axis of the Poly's plane} END_pt.x := offsetX + 1; END_pt.y := offsetY; END_pt.z := offsetZ; {Transform the point clicked on the Poly to the document XY plane} v := RelativeCoords(pt, beg_pt, END_pt); Message('Vector:', v); {SetPlanarRefIDToGround(selectedPoly);} {Draw the Rect at this point on XY plane} Rect(v.x,v.y,v.x+100, v.y-100); {Transform the Rect back to the plane which the Poly is drawn on by setting it's Entity matrix to be the same as the Poly's} {If you comment out the next line you will see the Rect drawn at the correct location relative to the Document Origin. But when I set the entity matrix it gets transformed to the correct plane but with an 'incorrect' (compared to what I expected) offset relative to the Poly} throwawayBool := SetEntityMatrix(LNewObj, offsetX, offsetY, offsetZ, rotationXAngle, rotationYAngle, rotationZAngle); {Message('Status: ', throwawayBool);} END; RUN(Main); Why when I set the entity matrix of the Rect does it not get transformed back to the original xyz point from the TrackObject call? Can anyone explain what I have misunderstood here? Thanks, W
-
Could someone explain what the coordinates are that GetPt returns? Are they screen plane coordinates, active working plane coordinates, layer plane coordinates or document XY plane coordinates? OK I experimented a bit and it seems they are active working plane coordinates but the working plane doesn't seem to be preserved between the call to GetPt and the call to Rect. Setting the plane ref of the Rect to be the same as a previously selected Poly is on a hiding to nowhere as there doesn't seem to be a way to get the origin of the plane? Is there any way to store the working plane that exists while the GetPt function is executed so that I can draw to it or is this a fundamental limitation of Vectorscript? I think I will probably just give up if I have to go and relearn 3D matrix maths and reconstruct everything from first principals...
-
Hi, I'm using this to try and draw a rect on a 3D plane. Note that the variable selectedPoly contains a handle to a 2D poly on a 3D plane. ... GetPt(pX, pY); Rect(pX, pY, pX+100, pY-160); planeRef := GetPlanarRef(selectedPoly); SetPlanarRef(LNewObj,planeRef); ... What I had hoped would happen is that the GetPt function would return the coordinates of the mouse click on the plane the poly is drawn on, as this is highlighted using automatic working plane when I make the click, and that I could draw a rectangle at these coords and then set it's plane ref to be the same as the poly. Having done this I would expect the newly drawn rect to appear under the cursor which it doesn't, the rect is at least aligned to the same plane as the poly though. Does GetPt always return a point on the document XY plane regardless of the active working plane? How does SetPlanarRef transform the rect from the document XY plane to the plane the poly is on?
-
Ahh, OK so the compile error is because I'm not using the result of the function. I didn't understand the error message. Thanks
-
Here's my code edited down. I'm trying a different drop in function here but still having problems. PROCEDURE Main; VAR selectedPoly, lastSlate:HANDLE; pX, pY, pZ, outZ:REAL; pt:POINT; FUNCTION CheckObjCallback(h : HANDLE) : BOOLEAN; VAR objType:INTEGER; BEGIN {GetTypeN Returns the type index of the referenced planar or screen object. Rectangle 3 RECT. Polygon 5 POLY} objType := GetTypeN(h); IF ((objType = 3) OR (objType = 5)) THEN CheckObjCallback := true ELSE CheckObjCallback := false; END; BEGIN {TrackObject Interactively, including highlighting, allows the user to select one object meeting the specified criteria} TrackObject(CheckObjCallback, selectedPoly, pX, pY, pZ); Message('Found Poly: handle:', selectedPoly, ' Point clicked: ',' x: ', pX, ' y: ', pY, ' z: ', pZ); pt.x := pX; pt.y := pY; GetZatXY(selectedPoly, pX, pY, outZ); END; RUN(Main); This wont compile, here's the error: Line #25: GetZatXY(selectedPoly, pX, pY, outZ); | { Error: Cannot use a FUNCTION name here. } { Error: Expected a RUN statement at the end of the scirpt } Also, I have no idea from reading the documentation if the GetZatXY function will operate on a 2d poly drawn on an arbitrary 3D plane. The concept of 2D stuff floating around in 3D space on planes is quite confusing why isn't it all just 3D? If i want to make a pure 3D object aligned with a 2D poly drawn on an arbitrary 3D plane whats the best way to do this? Do I have to convert the Poly to 3D, transform it to the document origin plane, do my stuff (I want to tile the poly with 3D 'tiles') then transform everything back into position?, what's the easiest way to apply a transform to some geometry? Can I draw 2D rects to the 2D plane that the poly is on then extrude these and rotate about a point on the extrude using a transform relative to the 2D plane? (thats roughly what I do if I was coding this in sketchup). Thanks for any help/advice you can give Will
-
Get rid of design layers and call them Design Volumes or Design spaces or something. Get rid of classes and replace them with Tags, the difference being that multiple Tags can be attached to a single object. So I can tag something as being for example 'Floor 3', 'Cladding', 'Type D', 'Fudge brown'. Design spaces ('layers') could then just be spatial queries; so you could say 'I want to see everything within these clip volumes'. Where an object is clipped you would have some edit handles where these make sense so you could still move walls around in plan. Saved views save which clip volumes, tags etc are active. You should be able to activate multiple saved views at the same time. Example Fire fighting stair saved view + 3rd floor reception saved view for while I'm looking at escape lobbies, for example. Or say add the views for chiller circuit + cooling towers + chiller plant. Boom! PS, Compromise for 2D old timers; design layers could still be around somewhere as additional sheet annotation layers or something. PPS I think Solidworks can probably do all this already.
-
Hi, I just noticed that if you look at the vectorscript guide online here http://developer.vectorworks.net/ there are lots of extra Vectorscript functions that don't appear to be documented in the Vectorscript docs in the /Vectorworks 2013/VWHelp/Vectorscript reference folder. Here is an example: http://developer.vectorworks.net/index.php?title=VS:RelativeCoords at the bottom of the page is a little note saying 'Drop in function'. I can't work out how to call them? I just get compile errors. I've been googling this for about an hour and I can't find anything about what this means and how to use them... Can anyone help? W
-
Improved scripting support
Will replied to Will's question in Wishlist - Feature and Content Requests
DWorks, .NET is tricky on the mac. Rhino has a .NET API but they haven't managed to port it over to the mac version of Rhino yet because they can't work out how to do it. Probably it would be simpler to interface with one of Python or Ruby or another open source language. W -
Improved scripting support
Will replied to Will's question in Wishlist - Feature and Content Requests
Miguel, I'm just learning Vectorscript so I'm grateful for any feedback, here's the updated script based on your comments. PROCEDURE Main; VAR className :STRING; index, classCount : LONGINT; numTest, uscoreTest: BOOLEAN; returnedNumber :REAL; BEGIN classCount := ClassNum; FOR index := 1 TO classCount DO BEGIN className := ClassList(index); numTest := ValidNumStr(Copy(className, 1, 2), returnedNumber); uscoreTest := Pos('_', className) = 3; IF ((numTest) AND (uscoreTest)) THEN RenameClass(className, Concat('Site-OS-',className)); END; END; RUN(Main);[/Code] I think you have to admit that it's still pretty clunky though. By the way, I noticed in your comment above that you call the Pos() function in the conditional. My script doesn't compile if I call a function in the conditional statement, I'm not sure why this is, seems like an unusual constraint. I tried googling for help on this but didn't get anything. Is this expected? Your point about the API being more important than the language is true, but if you look at the standard libraries for Ruby, Python, C# or whatever they provide lots of useful general functionality which Vectorscript is lacking, quite a lot of the Vectorscript API is replicating stuff thats available by default in the standard library of mainstream languages. In the long run, surely it would be less effort to deprecate Vectorscript and start afresh with only the API needed to provide CAD and BIM functionality? -
Improved scripting support
Will replied to Will's question in Wishlist - Feature and Content Requests
OK so here's a concrete example. I wanted to rename classes that started with two digits and an underscore. e.g. a class called '04_LANDFORM' would be renamed but 'Notes-Title block' would not. Heres the pascal code to achieve this in Vectorworks: PROCEDURE Main; VAR className :STRING; index, classCount : LONGINT; firstChar, secondChar : BOOLEAN; FUNCTION IsInteger(theChar:STRING):BOOLEAN; VAR asciiNum : INTEGER; BEGIN {ascii codes 48 to 57 correspond to 0 to 9} asciiNum := Ord(theChar); IF ((asciiNum > 47) AND (asciiNum < 58)) THEN IsInteger:= TRUE ELSE IsInteger:= FALSE; END; BEGIN classCount := ClassNum; FOR index := 1 TO classCount DO BEGIN className := ClassList(index); firstChar := IsInteger(Copy(className, 1, 1)); secondChar := IsInteger(Copy(className, 2, 1)); IF ((firstChar) AND (secondChar)) THEN RenameClass(className, Concat('Site-OS-',className)); END; END; RUN(Main); And here's the equivalent to rename layers in sketch up Sketchup.active_model.layers.each{|item| item.name = ("Site-OS-" + item.name) if item.name[/^\d{2}_/] } I didn't manage to recreate my swap tool yet because I haven't had time but I will do this eventually. -
Improved scripting support
Will replied to Will's question in Wishlist - Feature and Content Requests
C++ programming is too complicated to just pick up and use for quickly solving small problems. You need to program C++ as your day job for years to really be able to use it properly. It took me a couple of hours to create my first useful Ruby Sketchup script. The nice thing about using a modern language for scripting instead of Pascal is that there is masses of learning material on the internet and the debuggers, syntax checking, autocomplete etc is all done already and well supported by third party IDEs. Instead of using resources developing their own IDE for Pascal within Vectorworks, Nemetschek could supply plugins for Eclipse, Textmate, or whatever to enable features like syntax highlighting and autocomplete for the Vectorworks API. Ruby / Python are open source MIT style licenses so the source code can be incorporated into a commercial app. Tools such as SWIG can be used to automatically generate bindings to the Vectorworks C++ object model so it's not such a big a task to integrate one of these into VW. Cinema4d has a Python scripting interface, as does Modo, Rhino and Blender. Sketchup has Ruby. Solidworks, Microstation, Rhino and AutoCAD can all be programmed with any .NET supported language which means as well as VB you can use C# which is a bit more complicated than Ruby/Python but is still pretty nice to work with and has memory management and OOP. On the other hand Vectorworks is struggling along with this slow and clunky pascal thing which no-one programs in anymore because it stifles creativity. As to it just being a matter of syntax and know how. Well C++ is just a matter of syntax and know how. Assembly code is a matter of syntax and know how... Why would Apple have bothered to switch from PASCAL to C and Objective-C if Pascal was good enough. Why would Microsoft have bothered to create C# and the CLR if C++ was good enough. Once you have a modern flexible programming interface you can add features much more quickly (quite a few sketchup features are ruby plugins) and 3rd parties would be a lot more enthusiastic about developing for the VW cad platform. -
Improved scripting support
Will replied to Will's question in Wishlist - Feature and Content Requests
I don't know yet, I'm looking into it and I'll paste the code here if I get around to figuring it out. The point I was making was more about the constraints of a purely procedural language like pascal and the lack of modern scripting features like being able to write reusable modules, support for 'objects' (in the coding sense of the word) and 'duck typing' which makes scripts much easier to write. E.g. if ruby was fully supported, you would have access to the huge number of libraries (ruby 'gems'), so it would be very easy to access and manipulate web API's like the dropbox API. So it would be easy to write some code to issue drawings to an online service like dropbox. I think rhino has recently added the option of writing python code, but I've never used it. Neither autoCAD or microstation has particularly good or well documented scripting support though. I tinkered with visual basic in microstation and it was pretty painful. I found it easier to tinker with microstation in C# than VB. -
I'd like much better support for scripting. The ruby scripting API for google sketchup is light years ahead of the pascal scripting system used by VW. See here: https://developers.google.com/sketchup/docs/tutorial_geometry For example, here's a bit of code I wrote which allows you to right click on a component which you wish to exchange for another component and select the new component to use by simply clicking an instance of it in the drawing. Heres the code which makes the swap: def swap_components(theDef) ents = Sketchup.active_model.selection ents.each { |ent| ent.definition = theDef } end Heres the code which handles adding a menu option to the UI class SwapTool # The activate method is called by SketchUp when the tool is first selected. def activate Sketchup::set_status_text("Select replacement component", SB_PROMPT) end def deactivate(view) view.invalidate end # The onLButtonUp method is called when the user releases the left mouse button. def onLButtonUp(flags, x, y, view) ph = view.pick_helper(x, y) #pick helper deals with working out what you have clicked on ph.do_pick(x, y) entity = ph.best_picked return nil if not entity.kind_of?(Sketchup::ComponentInstance) #if the thing thats clicked on is not a component then do nothing. Sketchup.active_model.start_operation("Swap components") #tells sketch up that all the next commands will be one undo operation swap_components(entity.definition) # calls the swap_components method Sketchup.active_model.commit_operation #finishes the undo operation Sketchup.send_action("selectSelectionTool:") #tells sketch up to activate the select tool end def onCancel(flag, view) Sketchup.send_action("selectSelectionTool:") end end # class SwapTool As you can see it's pretty simple and concise compared to pascal. You could possibly use swig to generate bindings to your existing C++ classes automatically http://www.swig.org/ Perhaps you could also use QT for the user interface. http://qt.nokia.com/products/ I'd be happy with ruby, python, or any modern object oriented scripting language. Kind Regards, Will
-
Me too. About 5 crashes already today and at least 2 per day since I started working in 3D. Actually, working in 3D in VW is pretty disappointing so far. Is it really true that you have to buy Renderworks to get a usable shaded view that you can usefully work in? Also what's up with the weird 3D selection problems; it almost never selects the thing thats nearest to you, always something behind that you can't see which you weren't trying to hit. And the UI in VW is constantly flickering with little messages and things that highlight as you move the mouse around over the model, it's manageable in 2D but in 3D it picks up everything and makes me feel like having an epileptic fit. Is anyone else having problems with VW12 and 3D stuff? I'm using a site model and I wonder if it's too much for VW. Maybe I should cut and run and start using iRhino instead? What have others experienced? Sorry, that turned into a bit of a rant, it's after midnight now and I want to go to bed...
-
When I try to create a section viewport on a design layer, the viewport shows a plan? See attached screenshot. I can't work out how to make it show a section or elevation in this case. I'm sure I've followed the instructions in the help topic to the letter and it seems to work fine if I create a section on a sheet layer. Any ideas? Thanks Will