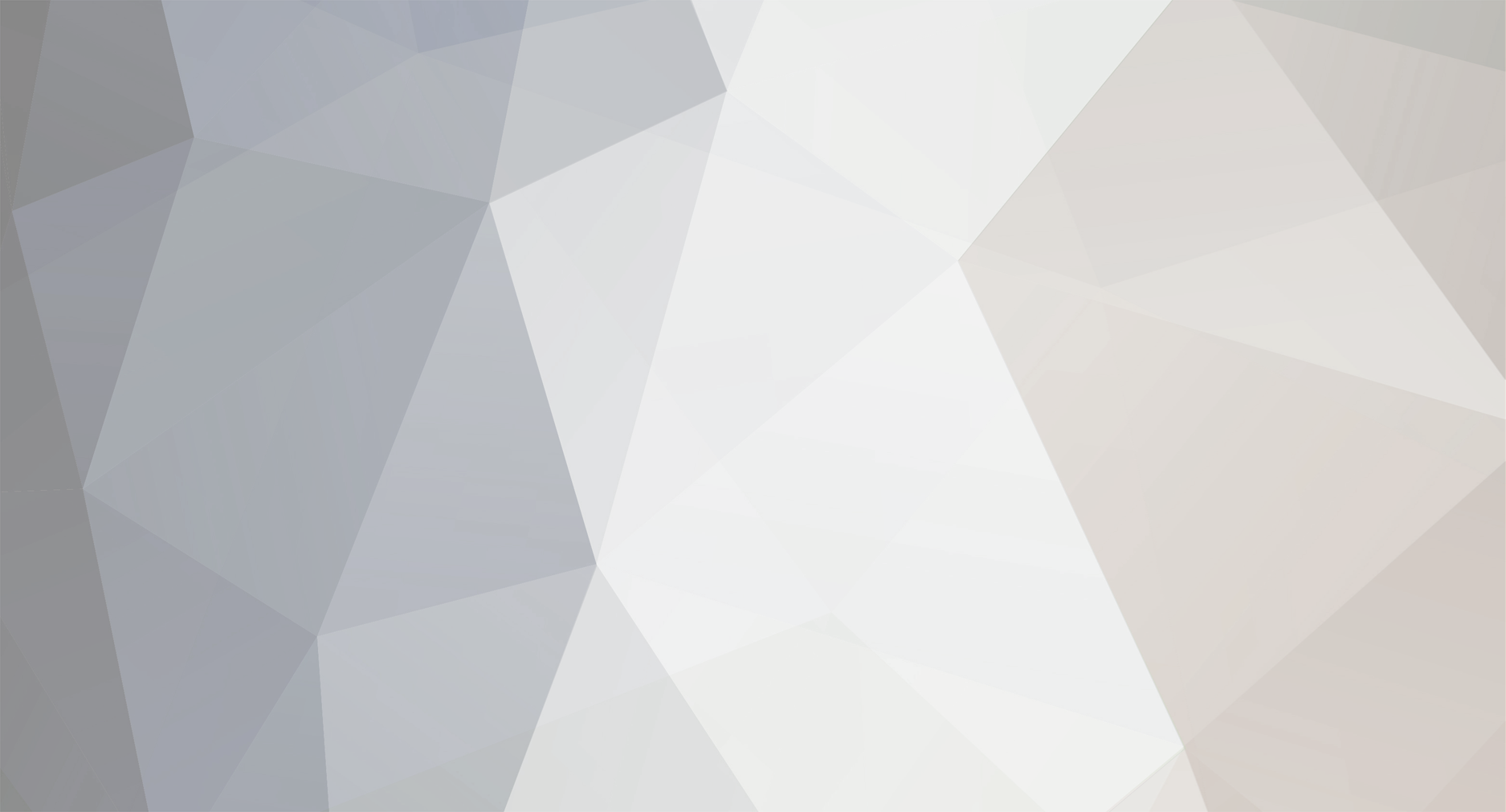
Jayme McColgan
-
Posts
196 -
Joined
-
Last visited
Content Type
Profiles
Forums
Events
Articles
Marionette
Store
Posts posted by Jayme McColgan
-
-
hey everyone,
I'm exploring a different side of VW plugins... i need to the ability to have the user draw a rectangles or polylines as a vso plugin. where should i start?
-
i ended up using importlib and it seems to be working fine.
import importlib importlib.import_module(module)
i do get warning of "pip is being invoked by an old script wrapper. This will fail in a future version of pip. Please see https://github.com/pypa/pip/issues/5599 for advice on fixing the underlying issue. To avoid this problem you can invoke Python with '-m pip' instead of running pip directly"
Can't seem to figure out a way to fix that but ill write back if i find a way to suppress that.
thanks to this forum for helping me
https://forum.vectorworks.net/index.php?/topic/52965-pil-python-image-library-for-vw-2018/
-
hey y'all,
some of my plugins require the user to pip install some modules into a Python Externals folder that lives the user folder. That part is working great but the user then has to restart VW in order to use them...
is there a way to reload VW without doing a full restart so it knows that those modules are now on the machine and can be used?
-
got it... had to use vs.GetVPCropObject() and vs.GetBBox() to get the location of the crop and move it with some math...
-
i know its probably staring me right in the face but i cant find how to take a new created Viewport (with a crop) and center that crop on a sheet layer
-
a down and dirty way is to look at the site-packages folder...
/Applications/Vectorworks 2022/Vectorworks 2022.app/Contents/Frameworks/Python.framework/Versions/3.9/lib/python3.9/site-packages
-
hey guys,
I'm working on a cad currently and its really slow... I'm wondering if theres a way to see something similar to a console log of what operations VW22 is doing so i can see whats hanging up the system. want to see if it's a plugin i built or what.
-
when you press a button it should return the number of the button pressed, you can then process the logic you need to from there. heres an example
import vs dialog1 = 0 def start(): global dialog1 dialog1 = vs.CreateLayout('Pixel Map Exporter', False, 'OK', 'Cancel') vs.CreatePushButton(dialog1, 5, "Im a button!") vs.SetFirstLayoutItem(dialog1, 5) result = vs.RunLayoutDialog(dialog1, Dialog_Handler4) return(result) def Dialog_Handler4(item , data): ### Init Setup if item == 12255: pass ### Finished elif item == 1: pass ### Canclled elif item == 2: pass ### Push Buutton Pressed based off the item number you gave it when building the dialog layout elif item == 5: vs.AlrtDialog(str(f"Pushed button! im item #{item}")) return None
-
1
-
-
yep that'll do it. and no i have another rabbit hole to dive down. lol thanks @klinzey
-
so i was playing around with the Speaker Array Tool and on the last tab they have a box with a table with resizable headers. what do you use to make that? i want to add a table to one of my plugins that uses dialog boxes? i've attached a picture of it.
I'm currently working with the vs.CreateListBox() but i don't think I'm on the right path...
-
my current workaround is building an external script that opens a powershell as administrator and then runs all the nessacry deleting/creating of files. not a perfect answer but it works.
-
ive found the built in ways to be very inconsistant... currently anytime i need to add a external python library i do a normal pip3 install and go find where it installed it which normally for me is in "/Library/Frameworks/Python.framework/Versions/3.8/lib/python3.8/site-packages".
i then copy and paste the installed libraries into a "Python Externals" folder in the user folder and its worked every time so far. i think i have 5-6 libraries in there.
-
@Myke i use the built in python functions to open files. i have a few plugins that are opening and creating files and I'm not using any of the VS functions to do that.
what exactly are you trying to do and i can whip up an example for you.
-
in my python externals folder i've added Pillow PIL and Requests (and the requested libraries for them).
EDIT:
well i guess i did'nt fully read the topic before posting... lol these 2 haven othing to do with vectors... sorry
-
On 1/12/2022 at 2:55 PM, JBenghiat said:
You can force an object to reset immediately by setting its object variable 1167 to true. However, I believe this only works if the object is SDK based, and I’m not sure about the stage decks.
In this specific case, you could always reverse engineer what the stage deck is doing and calculate your 3D points based on the stage deck’s parameters and location.
yeah ive been exploring this idea based on if its flipped or not but there are so many different variables to account for. ill post back if when i come up with a good way.
-
TLDR: i looking for a way to use vs.SetRField() on a selected PIO (in this case a stage deck) and have it refresh itself before continuing the script.
i'm working on a plugin that takes objects, converts them to 3dpolys, and bounces out their points to a file. I'm trying to cut down on the amount of points by simplifying the objects as much as possible before converting... lets say i have a "Stage Deck" object and i want to change its "Structure" field from "Legs-Basic" to "Simple" which really cuts down on its poly count. but when i try to use vs.SetRField() to change that field it doesn't seem to refresh the geometry... after the script runs you can see that the field was changed but the geometry is still the same.
i've tried the slu of resets and refresh commands and none of them seem to be helping. ive also tried changing the settings and then duplicating the object with vs.Duplicate() but vs.LNewObj() doesn't seem to be working with that.
-
-
I'm not in front of my computer to test but I often have to use the vs.refreshlightingdevice() command (I think that’s the name) when working with lighting devices.
-
using vs.CreateSymbolDisplayControl() and vs.UpdateSymbolDisplayControl() was giving me some unpredictable results when making 2D symbols so i ended up using vs.CreateImageControl2() and vs.UpdateImageControl3() and ended up making my 2d layouts with external libraries. getting much more predictable results!
-
a few issues things i encountered... i encountered these in both VW2020 and VW2022
- i think vs.BuildResourceList() is broken... the code below (which is just a portion of the whole script) return the correct num_list but the symfold_list wouldn't have a handles in it. i could also be using it wrong...
path = vs.GetFolderPath(23) + "MIG Symbols.vwx" symfold_list, num_list = vs.BuildResourceListN(92, path)
- i tried building a list using type 121 (Lighting Instrument - Symbols) but it always returned nothing.
-
here my version, might be able to be cleaned up some but it works for me.
import vs ### init List of all lights all_lights = [] ### init List of lights to ignore cur_lights = ["Viper", "Leko Body", "ETC Source 4 Body"] ### 121 = Lighting Devices ### 92 = Symbol Folders ### Loop though everything in a current symbol folder def start1(cur_handle): first_sym = vs.FInSymDef(cur_handle) for i in range(20): if first_sym.type == 16: add_Symbol(first_sym) first_sym = vs.NextSymDef(first_sym) ### Add lighting device to list def add_Symbol(current): hasRecord = vs.Eval(current,("R IN ['Light Info Record']")) if hasRecord: if vs.GetName(current) not in cur_lights: all_lights.append(vs.GetName(current)) cur_lights.append(vs.GetName(current)) return None ### main loop def start(): #path = vs.GetFolderPath(23) + "MIG Symbols.vwx" #symfold_list, num_list = vs.BuildResourceListN(92, path) symfold_list, num_list = vs.BuildResourceList(92, 0, "") vs.AlrtDialog(str(num_list)) for i in range(num_list): cur_handle = vs.GetResourceFromList(symfold_list, i) start1(cur_handle) vs.AlrtDialog(str(all_lights)) return None
-
yeah i got that. ideally i want to go though everything in the resource browser.
I'm starting to make some head room with vs.FSymDef() and others that go along with it. i understand i will have to deal with each type of symdef and loop though folders within folders as they pop up to get symbols within.
ill pick this back up tomorrow. going to sleep
-
so heres a slightly different question...
how do i loop through all symbols in the resource browser and build a list if a symbol has a certain record attached to it.
in this case i need to build a choice list for a dropdown in a dialog box.= based off symbols that have a specific record attached to it.
-
8 hours ago, MullinRJ said:
Quick answer: vs.ForEashObjectInList()
There is a nearly perfect example in the Function Reference.
Raymond
can't really seem to figure out how to do that for the items that live in the resource browser. I'm not trying to work on symbols that are placed in my drawing...
drawing a rect with a vso plugin
in Python Scripting
Posted
well would you look that! also its kinda a bummer that the rectangular tool is center based, very odd looking... after trying them all out the path based plugin will probably be my answer for now. ill have to look into what vs commands i need to call to set the path as a visible object on the screen. thanks for the help!