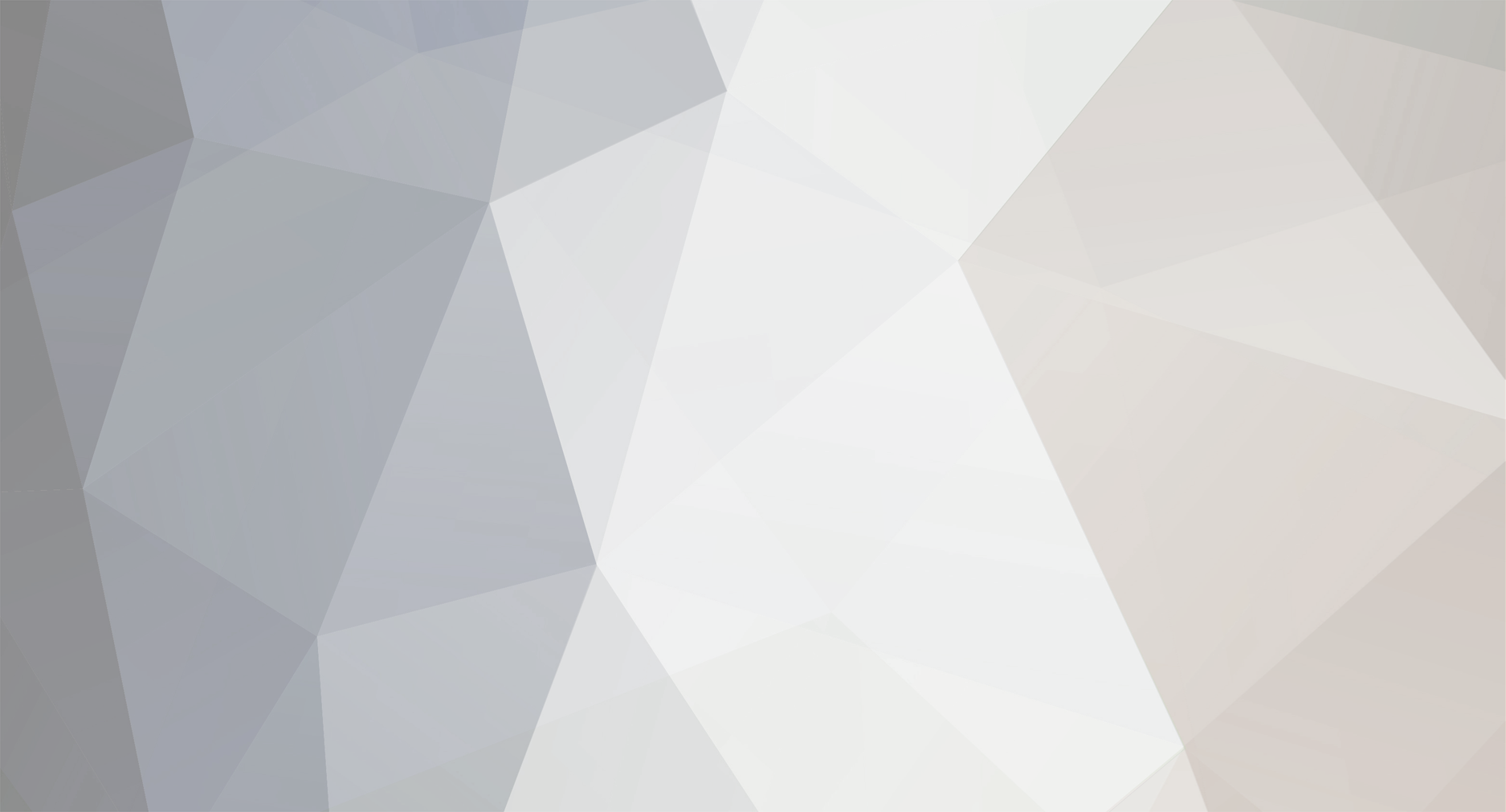
MullinRJ
-
Posts
2,007 -
Joined
-
Last visited
Content Type
Profiles
Forums
Events
Articles
Marionette
Store
Posts posted by MullinRJ
-
-
Hi Who,
???You're definitely on the right track. However, you need to calculate the angle you want to rotate from points 1 and 3 before you call the RotatePoint() function. This is most easily done with vector function Vec2Ang(). Since point 1 is the center of interest (and rotation) subtract it from point 3 and stuff it into vector V as follows:
V.x := x3 - x1;
V.y := y3 - y1;
AngleOfPt3 := Vec2Ang(V);???{ relative to point 1 }
The following script is not what you want, but it executes in a humorous way. Try it.
PROCEDURE yyy; VAR H :Handle; x1, y1, x2, y2, x3, y3, AngleOfPt3 :Real; V :Vector; BEGIN repeat if (MouseDown(x1, y1)) then begin moveto(x1, y1); x2 := x1 + 580; y2 := y1; closepoly; poly (30,#90,x2,#0,30,#-90); H := lnewobj; end; until (H <> Nil); repeat getmouse (x3, y3); V.x := x3 - x1; V.y := y3 - y1; AngleOfPt3 := Vec2Ang(V); RotatePoint (x1, y1, AngleOfPt3); redraw; until (MouseDown (x3, y3)); END; Run(yyy);
Since you are in a loop, each time through you will rotate your object again, so your script has to remember how much it rotated the object the last time through and subtract that off for the current pass through the loop. This example should be more to your liking.
PROCEDURE xxx; VAR H :Handle; x1, y1, x2, y2, x3, y3 :Real; AngleOfPt3, lastPt3Ang :Real; V :Vector; BEGIN repeat if (MouseDown(x1, y1)) then begin moveto(x1, y1); x2 := x1 + 580; y2 := y1; closepoly; poly (30,#90,x2,#0,30,#-90); H := lnewobj; end; until (H <> Nil); lastPt3Ang := 0; repeat getmouse (x3, y3); V.x := x3 - x1; V.y := y3 - y1; AngleOfPt3 := Vec2Ang(V); RotatePoint (x1, y1, AngleOfPt3 - lastPt3Ang); { rotate by delta angle } lastPt3Ang := AngleOfPt3; redraw; until (MouseDown (x3, y3)); END; Run(xxx);
HTH,
Raymond
-
I refrain from trusting bibles and rely more heavily on observation. I'm from the school of trial and error. Bibles are full of good intentions, but historically are not factual.
Without knowing what you are trying to do, I cannot offer sound advice on which path to take. There's usually more than one way to approach each problem, so if this command doesn't suit your needs, others might.
The whole point of GetClosestPt ,as described in the function ref guide, is that it returns the handle of the closest object to the user point. In your example you seem to be forwarding the handle (obj) to GetClosestPt when in fact it should be returning a handle?????You are right, the bible does say that, but the code acts differently. If you want it to work as advertised, you should file a bug.
In the meantime, describe what you are trying to do and maybe someone out here can suggest a decent solution or workaround.
Raymond
PS - In your search for truth, keep good notes.
-
Mattie,
???I don't think it ever worked the way you want. It would be nice if it did. Perhaps if you temporarily open up your snap radius to as large as it will go before clicking then resetting it after you get your object, it might act more like you desire.
???Use pref numbers 1009 and 1010 with GetPref() and SetPref(). If you need help with this, write back.
Raymond
-
Hi Matie,
Two things, you are missing a few lines from the example that is posted in the VS Function Reference. The full script is (with one line added):
PROCEDURE GetClosestPtExample;
VAR
???obj :HANDLE;
???ptX, ptY :REAL;
???index :INTEGER;
???containedObj :LONGINT;
BEGIN
???GetPt(ptX, ptY);
???obj := PickObject(ptX, ptY); { you need this line }
???GetClosestPt(obj, ptX, ptY, index, containedObj);
???SetPenFore(obj, 65535, 0, 0);
???{ the following line shows the values of your variables at the end of the script }
???message('obj= ', obj, ' X= ', ptx, ' Y= ', pty, ' ', index, ' ', containedObj);
END;
RUN(GetClosestPtExample);
Second, when you run the script and click in the drawing you have to be close enough to an object for it to be selected; the same as you would if you were trying to select it manually. Clicking in an open region will return zero in your "obj" variable.
HTH,
Raymond
-
There is no link from a Symbol Definition (where you are) to symbol instances. You will have to search the document to find any instances. Use one of the ForEachObject...() commands. If it's out there you should be able to find it.
Raymond
-
Personally, I'd invest in a full keyboard. There's no need to shell out $50-$100 for a new one. I just bought a BT keyboard at a used equipment flea market for $10 on Saturday. It works very nicely. (I'm typing on it now.) Shop around - EBay, Amazon, etc. If you find one you like, you'll also have a spare for emergencies.
Bottom line: If you plan to use your computer a lot, you'll be using your keyboard a lot. Get one you are comfortable with.
Raymond
-
What happens when you import in 2008, save the file and open it in 2010?
Raymond
-
Use ForEachObjectInList() and pass a handle to the first SymbolDef in the Symbol List.
Add the following line and create a similar function that filters object by class so that you don't change everything. This is in addition to the code you already have.
ForEachObjectInList(ChangeNameInSyms, 0, 2, FSymDef); { ALL objs, DEEP, SymDef List }
HTH,
Raymond
-
oldList: ARRAY[1..kTOT_CLASS] OF STRING:
newList: ARRAY[1..kTOT_CLASS] OF STRING:
Hi Andrew,
???Both of these lines end in COLONS and should end in SEMICOLONS. They're typos. Change them as follows and you should be good.
oldList: ARRAY[1..kTOT_CLASS] OF STRING;
newList: ARRAY[1..kTOT_CLASS] OF STRING;
???The remaining errors are only due to the pseudo-code. Put in your specifics and it should compile.
???One thing to note when a compiler kicks an error, sometimes the error is on the immediately preceding line. If you can't find an error on the one reported, look at the previous one.
Raymond
-
VW does not have a setting for LINECAPS. Would that it did.
I control all of my linecaps outside of VW, but this is not a viable solution for the masses and requires a LOT of forethought when trying to draft with multiple trimmings on the ends of Lines, Arcs, Polylines and Polygons.
File a WISH. It's a major shortcoming of minor feature that should have been implemented long ago.
While you are waiting, you might try to script a solution, or pay someone to do it for you, that will convert centerlines to outlines. Good luck.
Raymond
-
Yes, Reshaper is written in VectorScript for Vectorworks, so it will work (should work) on both Mac and Windows platforms equally. That has been one of the challenges in its development, to make it work the same on both as there are minor differences in the way VS performs on the two machines (mostly in the Modern Dialog calls). The sad thing is when I get it right, nobody notices. :-p
If you'd like to try it, send me the last 6 digits of your VW serial number and I'll send you a copy and a DEMO KEY that will enable Reshaper for 30 days. Also, tell me what version of the software you want it for. From your signature I'd guess you'd want it for VW 2009, but I have versions of Reshaper working from VW 11 to VW 15 (2010).
Raymond
PS - Oh yeah, an address might help. info@siriussolutions.net
-
Hi Brian,
???Set the page size with the Page Setup menu under the File menu.
Raymond
-
Digi'M,
???You are almost there with your VW generated script. Unfortunately, VW does not take it to the next step, which is to set up a loop to check multiple conditions. For that, you have to do a little scripting yourself.
???Luckily for you, I have one here. The trick that makes this work is knowing how to pass a <criteria> expression to one of the VS calls that require it for input.
???This is the part that is not easily found in the documentation:
VAR CRITERIA :STRING;
and you can build your own string and pass the result to a waiting VS command for further processing. If the string is malformed it may compile but you will either get a runtime error or no action at all.
???In your case I built the string from 3 pieces and glued them together with the concat() function. The first and third pieces are static text, while the middle part is the number you want to insert as a wildcard.
PART 1) eotroads_
PART 2) #???( a number from 1 to 999 )
PART 3) Rec.Class = 5
???When assembled, it will look like this (when # = 12): eotroads_12Rec.Class = 5
PROCEDURE SelectByRec; { Select objects that match the criterion "eotroads_###.Class = 5", } { where ### = 1 to 999. } VAR I :Integer; S :String; BEGIN DSelectAll; for I := 1 to 999 do begin S := concat('eotroads_', I, 'Rec.Class = 5'); { criterion in string form } SelectObj(S); end; END; Run(SelectByRec);
???I'm not sure what you are doing, but Pat's first comment might make your life easier, if you can change your record strategy. Tell us more about what you are doing and someone out here mighgt have some good ideas on how to use the software better.
HTH,
Raymond
-
I use nested includes extensively in Reshaper. Fifty-five files, three levels deep; No problem. I don't know if this is necessary, but I use the full file path name, and I also use the Windows path name delimiters "/" for cross platform stability.
Raymond
-
There is no command to FLIP objects by handle. There shoiuld be, but there is not. The way you are doing it is the only way I know of to do it, except as Miguel mentioned, draw it flipped if you can.
Raymond
-
Is there a possibility to have ()- or probably other special symbols in parameters name?
I believe you can use the Underscore character "_" (Shift-Minus) in parameter names.
Raymond
-
Hi John,
???You will have to set up a loop to read every record until you find the one with:
NoBearings=2
Use the POS() function to identify it and exit your loop. Then read the next two records and dissect each one accordingly.
Have fun,
Raymond
-
Thanks, Ed.
That site has a diverse, a very diverse, collection of videos. Thanks for sharing.
Raymond
-
Hi John,
I do this a lot. Here is a sample script. You'll have to add a loop and a READLN() to import multiple records.
HTH,
Raymond
PROCEDURE ParseIt; { Copyright 2010 Raymond J. Mullin } CONST Comma = ','; VAR S, T :String; param1 : Integer; param2 : Real; param3 : String; param4 : Boolean; function GetParam (var S :String; Delim :Char) :String; { Return the first substring of S up to the Delim character, } { and delete the substring from S. } Var DelimPos : Integer; Begin DelimPos := pos(Delim, S); if (DelimPos > 0) then GetParam := copy(S, 1, DelimPos-1); delete(S, 1, DelimPos); end else GetParam := ''; End; { GetParam } function UpperStr (S :String) :String; { Return UPPER CASE of String S. } Begin UprString(S); UpperStr := S; End; { UpperStr } BEGIN S := concat(7, Comma, 15.2, Comma, 'Texty Stuff', Comma, 'True'); { test string } T := S; { a copy of S } S := concat(S, Comma); { add a delimeter to the end of S if one is not already there } param1 := Str2Num(GetParam(S, Comma)); param2 := Str2Num(GetParam(S, Comma)); param3 := GetParam(S, Comma); param4 := UpperStr(GetParam(S, Comma)) = 'TRUE'; message(T, ' ---> ', param1, ' ', param2, ' ', param3, ' ', param4); END; Run(ParseIt);
-
This is not exactly what you want, but if you click in the drawing before a paste operation (not Paste In Place) the object(s) on the clipboard will paste at that new location, but the BBox center will be the relative insertion point. You may have to move it a little after pasting, but at least it won't paste across the drawing somewhere.
Another feature you can use is the Symbol Pickup Mode, third mode of the 2D Symbol Insertion tool. Select the Eyedropper Mode, click on a symbol and now that symbol is the active symbol. You are automatically returned to the Place Symbol Mode or Wall Insertion Mode, whichever was the mode you were in previously.
Use of the U key to toggle this mode is clunky as it always returns you to the Wall Insertion (WI) mode. Since you have to toggle through the WI mode to get to the Pickup mode the WI then becomes the last mode you were in. This interface still needs some work, but if you click instead of toggle the mode buttons, it will save you from having to search through a long list of symbols in the Resource Browser if the symbol you want is sitting right in front of you in the drawing.
Oddly, this mode is missing from the 3D Symbol Insertion tool. I haven't tried using the 2D Symbol Insertion tool (in Pickup mode) on 3D Symbols. It may work, so you might want to try it, but it suspect it won't snap to XY & Z. Still, it's worth a try.
Raymond
-
Thanks Pat,
???I've got it now. The Checkbox state is implied. When it's disabled the Layer Scale value is negative, although it doesn't show to be negative, just grayed. When it's enabled, the value is positive. Seems easy when you know the answer.
SetPrefInt(540, -GetPrefInt(540));
will toggle the checkbox and retain the Layer Scale value. I'll add a note to my PDF and repost it.
Raymond
-
Hi Pat,
???Pref 540 is the field to the right of the preference I am looking for. It's a PrefInt. I am looking for the boolean pref number that controls the checkbox. I don't believe there currently is a number for that, based on a script I wrote that compares all pref values before and after an event, in this case, the manual toggling of that check box. Nothing changes when that checkbox is set or cleared.
???The same is true of the other two DPI values under the Resolution Tab, BUT, if you do find something that escaped me, please let me know and I'll update the PDF's.
Thank you,
Raymond
PS - Don't spend too much time on it. I already compared every Integer, Longint and Real preference value from 0 to 32767. Not even a nibble.
-
The short answer is - Upgrade. Interactive Settings, which include being able to set a ton of cursor and highlighting colors, were introduced in VW 2009.
To adjust the "pickbox" size in VW 12, change the Snap Radius in VW Preferences > Edit Tab. it doesn't show any differently on the screen, but it does affect how close you need to be to snap.
Raymond
-
Here are two more annotated preference dialogs; VW Document Preferences and Unified View Options. The latter was posted in an adjacent thread but is also posted here to keep the bundle close knit.
In the Document Preferences dialog, there are three items where I did not find a preference number, despite searching the entire list:
???Display Tab > Hide wall components when layer scale <= 1: _____
???Resolution Tab > PDF Export (Quartz Only): _____ DPI
???Resolution Tab > Printing: _____ DPI
Should anyone know how to read or write these values, please let me know.
Raymond
Toggle autosave on and off
in Vectorscript
Posted · Edited by MullinRJ
To toggle autosave, use this one line script:
???setpref(24, not getpref(24));
To quickly find any constant that is used for a standard preference, go to: VW Preference Constants
Raymond